Summary
Salable nosql, schema less, database.
Interacting with database with CLI, shell, using mongosh
docker exec -it mongo mongosh
# to see databases
show dbs
# to select one db
use tfarraj-dev
# to see tables
show tabels # or show collection
# to see table columns by priniting one record
db.videos.findOne()
Mongoose: object modeling
Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js. It manages relationships between data, provides schema validation, and is used to translate between objects in code and the representation of those objects in MongoDB.
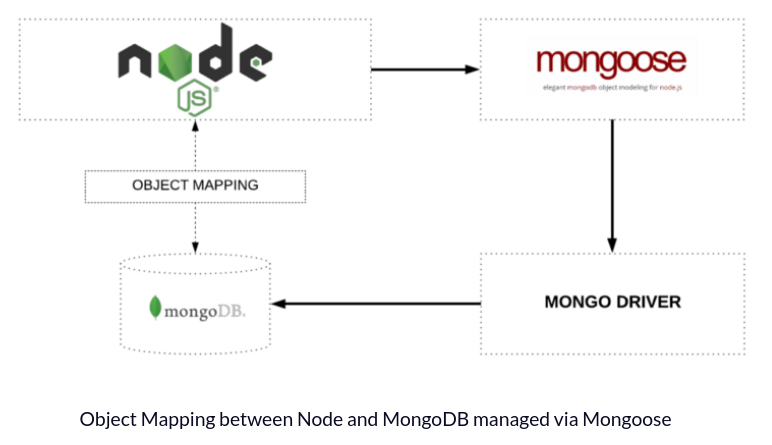
migrations
Common
- Both of these tools use mongodb feature to do the migrations.
- Both stores migration state in the database.
-
- use table or collection name to implement migrations.
- more popular: 232 commits, 618 stars and 14 contributors, used by 2.3k users
-
- mongoose models can be used in migrations
- less popular: 98 commits, 220 stars and 10 contributors, used by 1k users
Conclusion:
-
why use migrate-mongo?
- popular
- straightforward
- just manages the state of migrations, migrations are done using native mongodb features
-
why use migrate-mongoose?
- The only advantage of using migrate-mongoose over migrate-mongo is the features that allows using mongoose model at the expense of adding it own abstraction layer.
- The migrations can also be implemented without using mongoose model, similar to migrate-mongo.
- My personal preference is migrate-mongo, unless it makes it easier to deal with migrations using models.
migration examples
-
migrations samples migrate-mongo:
-
rename:
module.exports = async up(db, client) await db.collection('videos').updateMany( , $rename: content: 'game' , ); , async down(db, client) await db.collection('videos').updateMany( , $rename: game: 'content' , ); , ;
-
add property:
module.exports = await db.collection('videos').updateMany(, $set: tags: [] ); , async down(db, client) await db.collection('videos').updateMany(, $unset: tags: null ); , ;
-
-
migrate-mongoose
async function up() await this('user').create( firstName: 'Ada', lastName: 'Lovelace' );
notice the difference here “this“ instead of “db.collection” to access tables or collections.
-
using model and mongoose ODM
import User from '../models' async function up() await User.create( firstName: 'Ada', lastName: 'Lovelace' ); // OR do something such as const users = await User.find(); /* Do something with users */
-